Software has become the foundation of nearly every business operation. Whether it’s a mobile app, enterprise software, or a cloud-based platform, the demand for reliable and high-performing systems is higher than ever. However, developing functional software isn’t enough. What differentiates successful products from those that fail is how well they are tested and maintained.
That’s where quality assurance (QA) and software testing come into play.
This article explores the best practices in software engineering with a focus on quality assurance and testing — critical components for delivering robust, scalable, and user-friendly applications.
Fundamentals of Software Engineering
Software engineering is the disciplined, engineering approach to software development. It involves systematic processes, methodologies, and principles that ensure the creation of efficient, scalable, and maintainable software products. These fundamentals include:
- Software development life cycle (SDLC): A structured process defining phases such as planning, design, development, testing, deployment, and maintenance.
- Requirements analysis: Understanding user needs and documenting specifications.
- Design & architecture: Structuring the software using design patterns, architecture styles (e.g., microservices, monolith), and modeling techniques like UML.
- Implementation: The actual coding phase uses programming best practices.
- Maintenance: Updating and fixing bugs post-deployment.
While these stages are essential, integrating QA and testing early in the SDLC can dramatically improve product quality and reduce technical debt.
Quality Assurance Techniques
Quality assurance (QA) is more than just finding bugs; it’s about preventing them. It’s a proactive process that ensures quality is built into the product from the very beginning.
Here are some key QA techniques:
1. Test-Driven Development (TDD)
TDD is a practice where developers write tests before writing the actual code. This ensures that the codebase meets defined requirements from the start. TDD improves code quality and supports continuous integration.
Benefits:
- Faster feedback
- Reduced defects
- Well-documented code behavior
2. Continuous Integration/Continuous Deployment (CI/CD)
CI/CD pipelines automate testing and deployment, ensuring code changes are validated and delivered swiftly. Automated tests (unit, integration, end-to-end) are executed every time a change is pushed to the codebase.
Benefits:
- Reduced integration issues
- Immediate defect detection
- Faster release cycles
3. Code Reviews and Pair Programming
Code reviews involve examining written code for potential errors or design issues. When combined with pair programming, this technique not only catches bugs but also enhances team collaboration and knowledge sharing.
Best Practices:
- Use checklists for consistency
- Focus on readability, security, and efficiency
- Encourage constructive feedback
4. Static Code Analysis
Tools like SonarQube and ESLint analyze code for quality issues without executing it. They check for syntax errors, security vulnerabilities, and adherence to coding standards.
Why use it?
- Early bug detection
- Enforces coding standards
- Reduces technical debt
5. Risk-Based Testing
Not all features require the same level of scrutiny. Risk-based testing prioritizes testing efforts based on the impact and likelihood of failure, ensuring critical features are thoroughly vetted.
Importance of Testing
Testing is a subset of QA, but it’s one of the most vital pillars of software engineering. While QA is process-oriented, testing is product-oriented, focusing on identifying actual defects in the software.
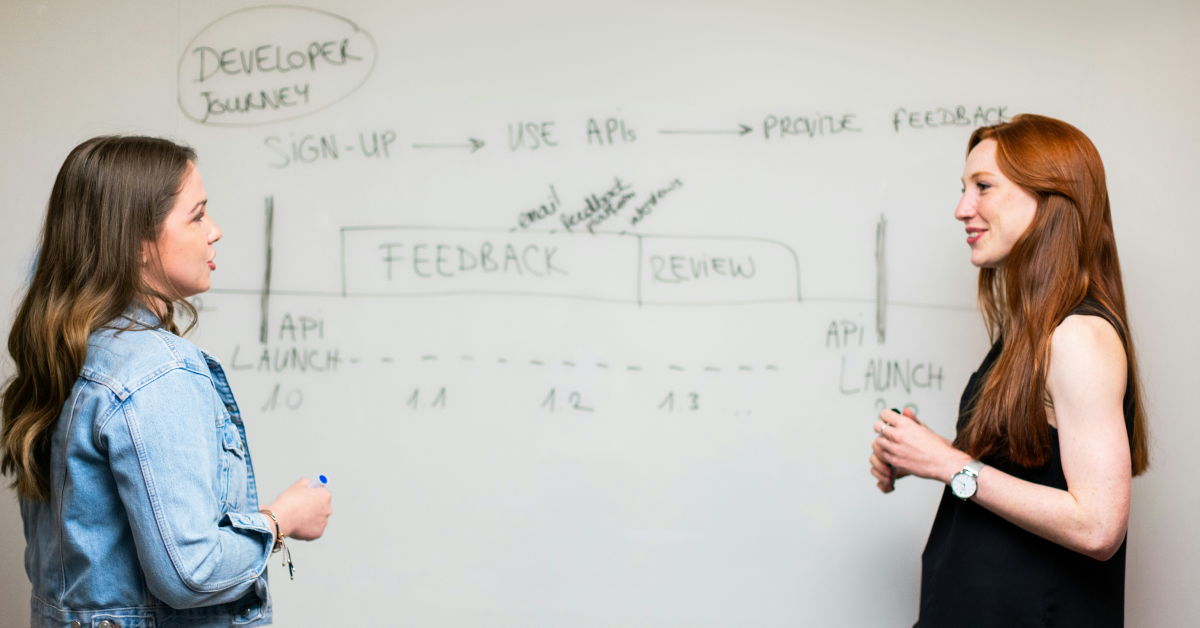
Types of Software Testing:
1. Unit Testing
The most granular level of testing where individual functions or methods are tested. This is often automated and forms the base of TDD.
2. Integration Testing
Ensures that different modules or services interact correctly. This can catch interface mismatches and data handling issues.
3. System Testing
This involves testing the entire system as a whole against the requirements. It ensures that the software works end-to-end as intended.
4. Acceptance Testing
Performed by end-users or QA to validate that the software meets business requirements. This is crucial before the product is released.
5. Regression Testing
As features evolve, regression testing ensures that new changes haven’t broken existing functionality.
6. Performance Testing
Assesses the software’s responsiveness, stability, and scalability under a load. Key types include:
- Load Testing
- Stress Testing
- Spike Testing
7. Security Testing
Evaluate the software for potential vulnerabilities such as SQL injection, XSS, or unauthorized access.
Best Practices in QA and Testing
The goal of QA and testing isn’t to achieve flawless software—perfection is a myth—but to minimize risks and deliver a reliable, user-focused product. Below are proven best practices, enriched with practical strategies and real-world context, to elevate software quality across the development lifecycle.
1. Shift Left Testing
Shift testing as early as possible in the Software Development Life Cycle (SDLC). Identifying defects during requirements analysis, design, or coding phases can save significant time and cost compared to addressing issues after deployment.
- Why It Works: Studies show that fixing a bug post-release can cost up to 100x more than catching it during development.
- How to Implement:
- Conduct code reviews with static analysis tools like SonarQube to catch issues before they propagate.
- Collaborate with developers to write unit tests during coding using frameworks like Jest or pytest.
- Involve QA in sprint planning to align test scenarios with user stories early.
2. Automate What You Can
Automation is a game-changer for repetitive, time-consuming tasks like regression testing, but it’s not a silver bullet. Focus on automating tests that deliver high value and stability.
- Why It Works: Automated tests can run 24/7, catching regressions faster than manual efforts, especially in CI/CD pipelines.
- Tools to Use:
- UI Testing: Selenium, Cypress, or Playwright for browser-based testing.
- API Testing: Postman, RestAssured, or SoapUI for backend validation.
- Unit Testing: JUnit (Java), pytest (Python), or Mocha (JavaScript).
- Pro Tip: Avoid automating flaky tests or one-off scenarios. Use a risk-based approach to prioritize tests with high frequency (e.g., daily builds) or critical functionality (e.g., payment processing).
3. Maintain a Robust Test Suite
A well-crafted test suite is the backbone of reliable QA. It should be comprehensive yet maintainable, balancing coverage with efficiency.
- Key Characteristics:
- Maintainability: Structure tests to minimize rework when code changes. Use page object models in UI testing for cleaner scripts.
- Comprehensive Coverage: Include positive (happy path), negative (error conditions), and edge-case scenarios. For example, test a login form with valid credentials, incorrect passwords, and SQL injection attempts.
- Modularity: Break tests into reusable components to avoid duplication. For instance, separate login logic from checkout tests in an e-commerce app.
- Documentation: Comment test cases clearly and maintain a wiki (e.g., Confluence) for test objectives and setup instructions.
- How to Achieve It:
- Use a test management tool like TestRail or Zephyr to organize and track test cases.
- Regularly review and prune outdated tests to keep the suite lean.
4. Test Environments Should Mirror Production
Testing in an environment that closely mimics production—down to hardware, network latency, and data volume—ensures results are reliable and reduces “it works on my machine” surprises.
- Why It Matters: Misaligned environments can hide critical issues, like performance bottlenecks or database compatibility errors, until deployment.
- Best Practices:
- Use containerization (e.g., Docker) and infrastructure-as-code (e.g., Terraform) to replicate production settings in staging.
- Seed test databases with anonymized production data to simulate real-world conditions.
- Simulate network conditions (e.g., latency, packet loss) using tools like Charles Proxy or Toxiproxy.
5. Monitor Post-Release
Testing and QA extends beyond deployment. Real-time monitoring and user feedback loops are critical for catching issues that slip through pre-release testing.
- Why It’s Essential: Users often encounter edge cases or performance issues under real-world conditions that lab testing can’t replicate.
- Tools to Leverage:
- Performance Monitoring: Datadog, New Relic, or Prometheus for tracking latency, CPU usage, and errors.
- User Behavior Analytics: Mixpanel or Amplitude to understand how users interact with features.
- Error Tracking: Sentry or Rollbar for instant alerts on crashes or exceptions.
- Actionable Steps:
- Set up dashboards to visualize key metrics like API response times or error rates.
- Implement feature flags to roll back problematic updates without redeploying.
6. Foster a Quality Culture
High-quality software is a team sport. QA teams can’t shoulder the burden alone—developers, product managers, designers, and stakeholders must all prioritize quality from ideation to delivery.
- Why It Works: When everyone owns quality, defects drop, and collaboration improves. For instance, Google’s “Testing on the Toilet” initiative embeds QA tips into developer workflows.
- How to Build It:
- Train developers in basic testing skills, like writing unit tests or using debuggers.
- Encourage product managers to define clear acceptance criteria in user stories.
- Hold blameless postmortems to learn from defects without finger-pointing.
- Celebrate quality wins, like catching a critical bug pre-release, to reinforce the mindset.
Conclusion
Mastering software engineering isn’t just about writing clean code or choosing the right framework. It’s about building systems that are reliable, maintainable, and deliver real value to users. Quality assurance and testing are the guardrails that help teams achieve this.
By adopting best practices like TDD, CI/CD, automation, and continuous feedback, teams can reduce risks, accelerate development, and build software that stands the test of time.
If you want to learn more, get all your insights from experts right here.